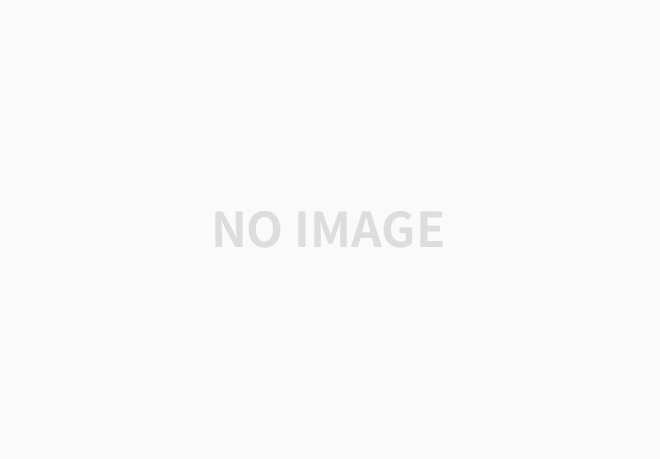
FloatingActionButton 이란 위 그림처럼 동그란 모양의 버튼을 말한다. (줄여서 fab라고 한다.)
해당 View를 사용하려면 아래 dependency를 추가해주자.
(Material로 추가해도 되나 design과 충돌 발생하기 때문에 하나만 추가해주자)
dependencies {
implementation 'com.android.support:design:28.0.0'
}
기본적인 사용 방법은 다음과 같다.
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/itemFab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:backgroundTint="#FFFFFF"
android:src="@drawable/create"
app:borderWidth="0dp"
app:fabSize="mini"
app:layout_constraintTop_toTopOf="@id/mainFab"
app:layout_constraintBottom_toBottomOf="@+id/mainFab"
app:layout_constraintStart_toStartOf="@id/mainFab"
app:layout_constraintEnd_toEndOf="@id/mainFab" />
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/categoryFab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:backgroundTint="#FFFFFF"
android:src="@drawable/category"
app:borderWidth="0dp"
app:fabSize="mini"
app:layout_constraintTop_toTopOf="@id/mainFab"
app:layout_constraintBottom_toBottomOf="@+id/mainFab"
app:layout_constraintStart_toStartOf="@id/mainFab"
app:layout_constraintEnd_toEndOf="@id/mainFab"/>
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/mainFab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:backgroundTint="@color/colorPrimary"
android:src="@drawable/add"
android:layout_marginRight="16dp"
android:layout_marginBottom="4dp"
app:borderWidth="0dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintBottom_toTopOf="@id/bottomNavigationView"/>
총 3개의 FloatingActionButton을 사용할거기 때문에 3개를 선언해주었다.
가장 큰 메인 버튼 뒤에 작은 버튼들이 숨어있는 형태다.
사용한 속성을 살펴보자면 다음과 같다.
속성 | 설명 |
backgroundTint | 배경 색상 |
src | 안에 들어갈 이미지 |
borderWidth | 테두리 |
fabSize | 버튼 크기 |
이 외에도 다양한 속성이 있는데
https://material.io/components/buttons-floating-action-button
Material Design
Build beautiful, usable products faster. Material Design is an adaptable system—backed by open-source code—that helps teams build high quality digital experiences.
material.io
위의 Material.io를 참고해보자.
이후 Activity에서 각 fab에 ClickListener를 구현해주자.
private var isOpen = false
val mainFabClickListener: View.OnClickListener = View.OnClickListener {
if(!isOpen) { // fab이 눌리지 않았다면
ObjectAnimator.ofFloat(itemFab, "translationY", -400f).apply { start() }
ObjectAnimator.ofFloat(categoryFab, "translationY", -200f).apply { start() }
isOpen = true
}
else{ // fab이 눌렸다면
ObjectAnimator.ofFloat(itemFab, "translationY", 0f).apply { start() }
ObjectAnimator.ofFloat(categoryFab, "translationY", 0f).apply { start() }
isOpen = false
}
}
val itemFabClickListener: View.OnClickListener = View.OnClickListener {
startActivity(Intent(this, ItemCreateActivity::class.java))
}
val categoryFabClickListener: View.OnClickListener = View.OnClickListener {
startActivity(Intent(this, CategoryCreateActivity::class.java))
}
mainFab(가장 큰 메인 버튼)을 눌렀을 때 차례대로 ObjectAnimator를 이용하여 좌표를 이동시켜주었다.
0f는 원래 있던 좌표 기준에서 0만큼 이동한 것이므로 원래 자리로 돌아오라는 코드이다.
해당 방식 말고 fab을 invisable로 해놓고 속성을 변경한다던가 애니메이션을 다른 방식으로 구현하는 등 다양한 방법이 존재한다.
-끝-
'Android > 이것저것' 카테고리의 다른 글
[Android] Retrofit, HTTP 통신을 위한 라이브러리 (0) | 2020.07.27 |
---|---|
[Android] Cleartext HTTP ~ bot permitted Error (0) | 2020.07.19 |
Fragment (0) | 2020.07.05 |
NotificationListenerService (0) | 2020.05.10 |
스플래쉬 (0) | 2020.05.10 |
댓글